In this guide, we will see the ultimate full stack development roadmap. You can use this roadmap for two purposes: become a full stack developer, and create a large and enterprise-grade web application. That is, if you are new to full stack development you can use this roadmap to see what to learn and in which order. And, even if you are not new to this, you can still use this roadmap to know which parts of your app to build first.
Full Stack Development Roadmap
This full stack development roadmap will present a component of your app and the technology you can use to create it. It will provide you with some details on why this component is best created at this stage (and not before or after), and some tips on how to create it. With minor tweaks, you can readapt this roadmap to be specific for your context and present it in form of a PowerPoint to your boss.
1. Backend (Node.js)
The first component you should create as part of your full stack development roadmap is the backend. The backend is the piece that contains all the logic of your app and that is running 24/7 on a secure server in the cloud, always accessible for the other part of the app (the frontend).
If your app is Instagram, the backend is the server (or pool of multiple servers) that holds all your pictures, messages, and followers. When you open the app on your smartphone, it just connects to the server and downloads that information. When you post a new picture, your smartphone publishes it to the server so that other users can download it and see it.
If your app is a bank, the backend is where you hold all the sensitive information such as the balance of user’s account, list of transactions, and so on.
Why backend first? Because backend can live without a frontend, but a frontend cannot live without a backend. The Instagram app on your smartphone is a frontend: if you have no connection, and thus can’t reach the backend, the app is pretty useless, isn’t it? Of course, a backend without a frontend has limited potential, but at least it can do something. If you are working with enterprise-grade apps, you may have external applications interact with your backend as an API – and you do not need a frontend for that, frontend is for humans.
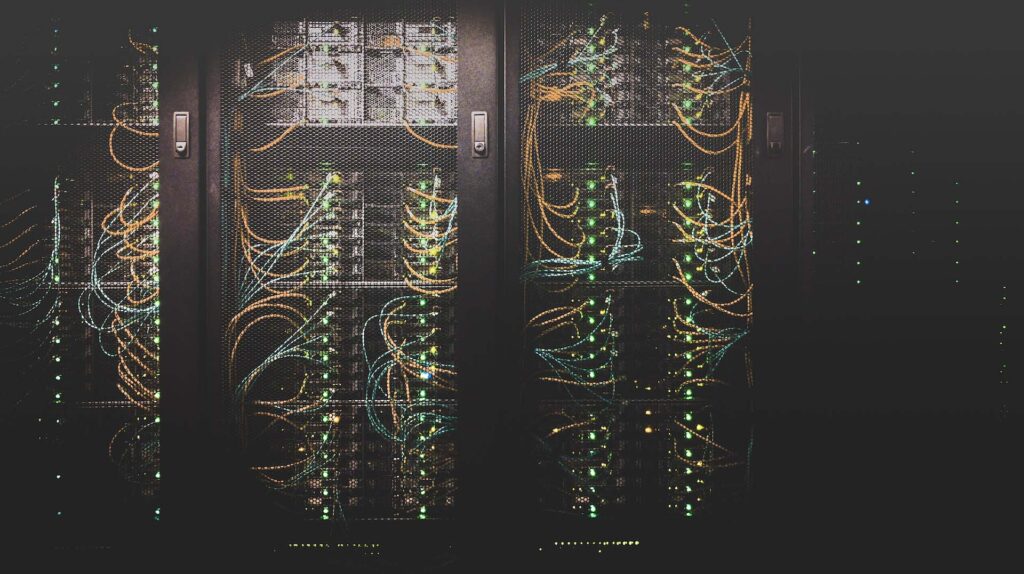
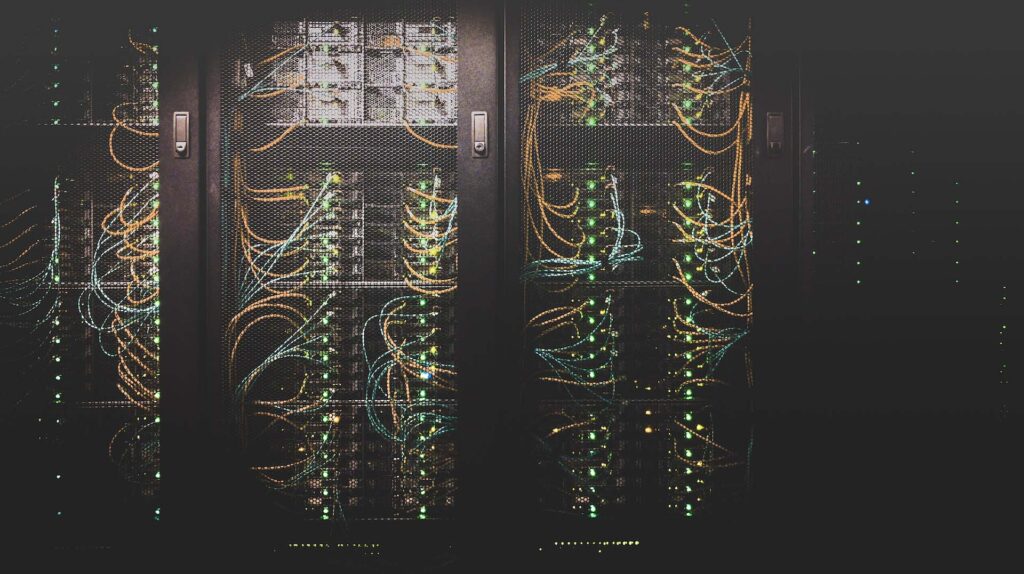
The backend is the most important piece of your app, and thus the first in the full stack development roadmap, because it contains all the logic and functionality of your app. And you need just one technology to make it happen: Node.js. This statement is not entirely correct, but the other technologies you will need are not specific to the backend itself.
If you want to create a backend for your app and you are first approaching Full Stack Development, you should learn the following technologies:
- HTTP – this is how frontend or external apps communicate with a backend, spend some time learning this. This is theory only, but will get you up to speed to understand what happens afterwards (you can do this in less than 4 hours).
- Node.js and npm – This is the server-grade JavaScript language and tools that you can use to create applications running on a server. Study this to understand how to implement all your business logic.
- Express.js – This is an additional package you can add to your Node.js app that will make it work as a backend, responding to HTTP requests. In other words, you already have the business logic, with this you can link some functionality to specific endpoints, or simply URLs that you call with some parameters to ask your backend to execute something.
- MongoDB or MySQL – Most likely, your app will need to store some data for later retrieval. You need a database engine for this. MongoDB is the easiest to start with, MySQL is also a good option if you want to use relational databases (better for banking applications in general).
- Git – This is a tool that allows you to store multiple versions of your code and keep track of what changes you make. It is also useful to collaborate, as you can see which developer introduces which changes to the code. Even if you work alone, Git is crucial because it allows you to restore your code to a previous point in time. If you don’t use it, you will shoot yourself in the foot at some point (note, Git is the protocol technology. GitHub.com is one of many services that offers a Git Server where you can store your code, but there are many more).
The advice part of this full stack development roadmap to new developer is to avoid going full throttle on each item and then move to the next. Just learn and do a little bit of the first, then move to the next, do a little bit there, and so on. You want to have an iterative approach. In other words, you don’t want to start using Git only when your backend is fully developed.
2. Frontend (HTML, CSS, Vue.js)
Once your backend is functional (or at least it has some of the functionalities needed), you can move to the next item in the full stack development roadmap: the frontend. The frontend is the piece of code that runs on the user’s device: the smartphone or the computer (even just inside the browser). Typically, the frontend is all about Graphic User Interface, GUI (or simply UI in most cases).
The goal of the frontend is to give the user a convenient way to interact with the backend. When you scroll Instagram, you just swipe your thumb on the screen and you automatically get the latest images. If there was no frontend, you would have had to write an HTTP GET request to download them from the web server – not very convenient.
There are many frontend technologies, mainly depending on where you want your app to run. If you want to create an Android app, you will need to create your frontend with Java and some Android-specific settings. If you want to create it for iPhone, you will need to learn Swift. However, the most common place to create a frontend is the web. You can create a frontend that runs in the user’s browser (Chrome, Edge, Firefox etc.), and that can thus run on any modern device. True, a native app on a smartphone offers a better experience, but we need to start somewhere.
There are really just three technologies to create a frontend: HTML, CSS, and JavaScript. However, we also have additional technologies that enable you to write code faster. But let’s go with order in our full sack development roadmap.
HTML, or HyperText Markup Language is the first you should learn. This is not really a programming language, but a “markup” language: it defines what is the role of each item on a web page. If you have a piece of text, you use HTML to define if that is a paragraph, a title, or the label of a button. Note that here we are not define any style or appearance for the content, we are just defining the role. It is about “calling things by their right name”.
CSS, short for Cascade Style Sheet, is where you add style. Since using HTML you were able to define what is a title and what is a paragraph, with CSS you can reference all the titles (or some) and define how they should look: size and color of the text, which font family to use, and so on. With HTML and CSS only you can create a beautiful (but static) user interface.
JavaScript comes to the rescue to make your app dynamic. This, unlike HTML and CSS, is a real programming language. The JavaScript that runs in a browser have some limitations, because the browser enforces security limits (you cannot access files on the user’s computer, for example). However, it has all the functionality we need to create a modern frontend: it can alter the HTML and CSS of the page, thus changing what is presented and how it is presented, and it can make calls to a backend.
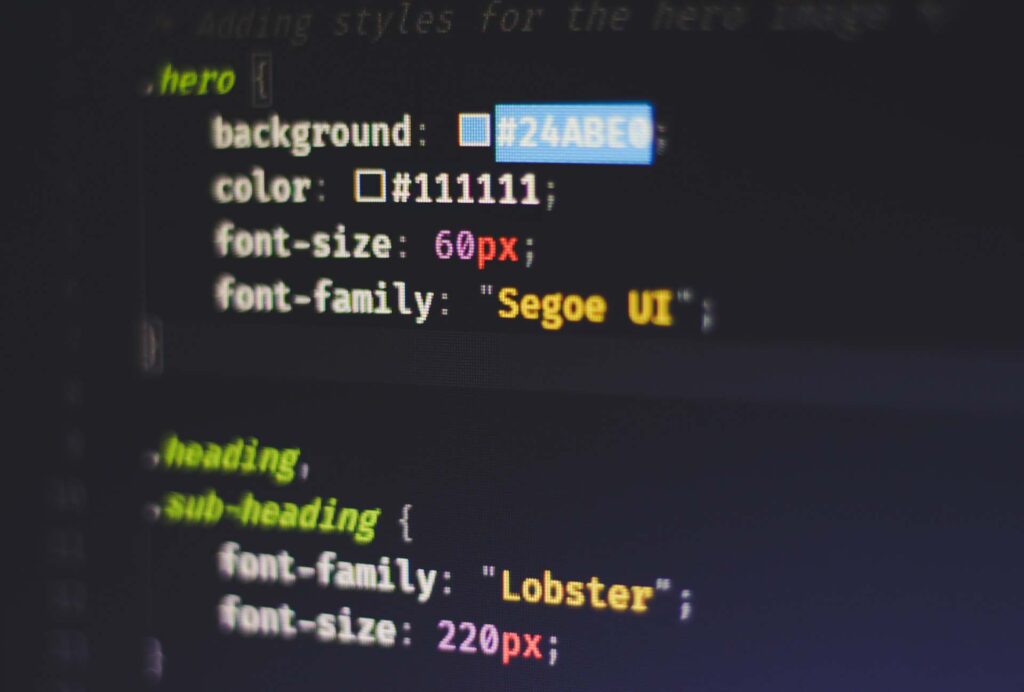
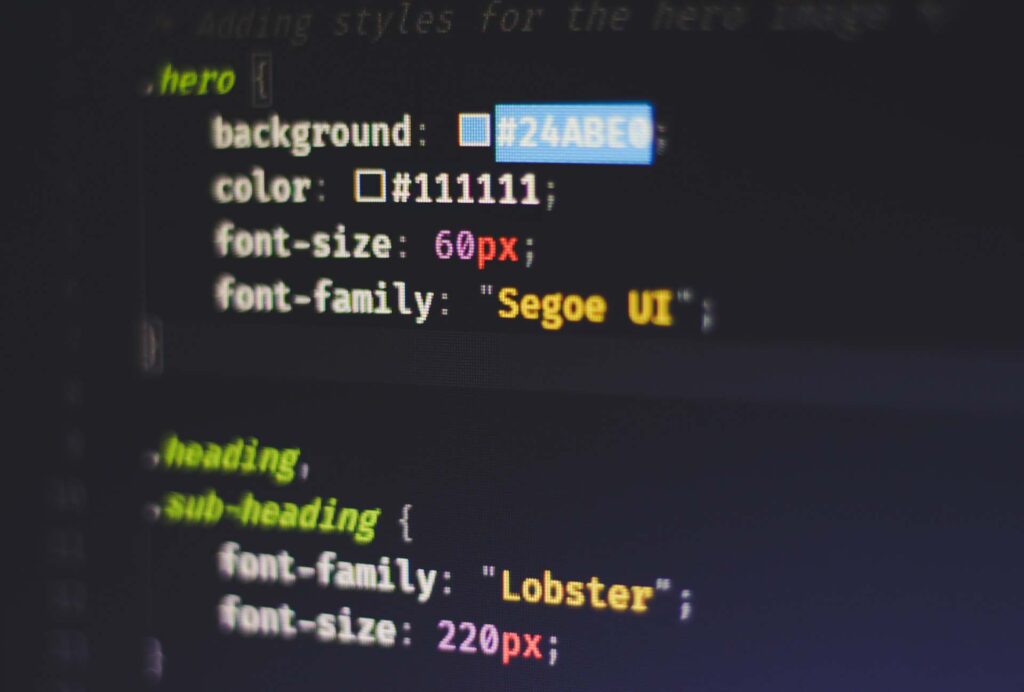
You thus use JavaScript to ask the frontend some content (for example, images if you are Instagram) and then alter the HTML of the page to make these images visible to the user.
Working with HTML, CSS, and JavaScript separately feels so early 2010’s. Nowadays, there is a much better way. We have Frontend JavaScript framework, that allows you to create modern frontend apps. React, Angular, and Vue are popular frameworks. I tend to prefer Vue.js because it has a much easier learning curve and can achieve the same results as the other frameworks, so this is what I have included in this full stack development roadmap.
3. Infrastructure Tools (AWS)
Disclaimer: at the moment of writing this article, I work as Program Manager for AWS. What you see here are my opinions only, and not the ones of Amazon. I also do NOT get any bonus, commission, or incentive if you use AWS for your app.
Imagine you now have your backend and frontend ready. You want to make them accessible and working for your users. To do that, you need to host your app in some sort of server. My advice here is to start simple and use AWS LightSail. This is a service on AWS that allows you to buy a server at a competitive price and avoid any unwanted charges, because it bundles everything you need beyond the server, such as data transfer capacity.
As your app grows, the first thing you want to do is to offload the frontend. Remember, the frontend runs on the user’s device, but the user needs to download this frontend from somewhere. Instead of making it available on the same server where your backend runs, you can place it in a S3 Bucket as a static website. This is a simple service that allows users to download files, and it feels like a website or app – except that you need to implement the business logic for the backend outside of S3 (keep them on LightSail).
Hopefully, your app will grow even further. At some point, getting larger and larger servers to run it is not scalable. You need to split your app across multiple servers. This is when you want to start learning about microservices, containers, Docker, and Kubernetes. Here, the logic is simple: each component of your app is like a standalone mini-server (a container), and all these containers interact with each other to make your app a reality. For example, the backend will only host business logic now, because the database is hosted on another container. Do not “oversplit” your app: communication between containers is an extra cost in terms of latency and time to load.
During all this journey, you will need to learn about DNS. That is the Domain Name Service, a protocol used over the Internet to associate names (such as ictshore.com) which IP addresses, unique numeric identifier of servers across the Internet. You need to learn about this because it is through a DNS configuration that you can make your server accessible at a specific domain name. It is yet through another DNS configuration that you can split the frontend and host it in S3, and you will still need DNS to containerize your app.
4. Deployment Tools (GitHub)
Our full stack development roadmap would not be complete if we did not mention CI/CD (Continuous Integration, Continuous Deployment). Those are abstract concept that means every time we push new code, that should be immediately integrated in the main code and deployed to the servers, so that users can access it.
In practice, this translate into having automated pipelines that, whenever a new change arrives, know how to deploy it to servers. Normally, this happens per steps: the first deployment is to a test server that can be broken, and only eventually we reach production server(s) where we have real users. But, in a real CI/CD process, all of this is automated.
GitHub.com offers a pipeline mechanism where you can do all of that, for free, if you host your Git repository there. Azure DevOps and AWS CodeCommit offer similar features.
Full Stack Development Roadmap in Summary
In summary, a Full Stack Development roadmap is about understanding which technologies are used and in which order to create a modern, stable, and useful web app. With this guide you should know where to focus the effort, and which parts of the app to approach first. We have more useful information about Full Stack Development in this other guide as well.